Friday, January 10, 2025
This is bananas: why you don't get arrays

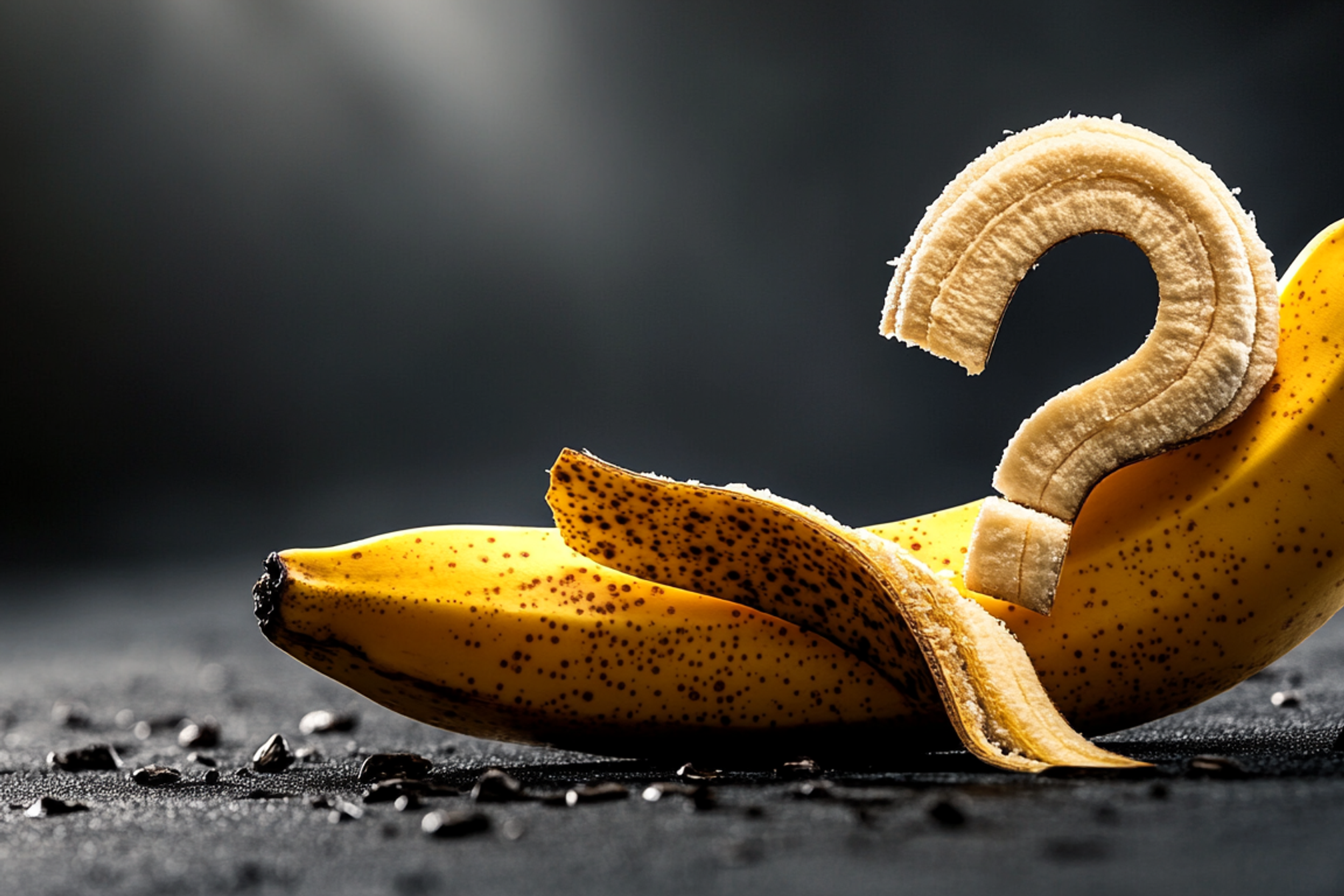
The array is among the first data structures you encounter when you first come in contact with coding. While they're relatively easy to wrap your head around on the outside, they get trickier on the inside when you think of methods like filter
, map
, or find
. I think I know why that is.
In every beginner tutorial, you will find arrays as part of the JavaScript basics and how to work with them. In fact, when you're learning programming there's no way around arrays; that's how basic they are. You will encounter them at some point.
Let's take the find
method and inspect how it works on the following array.
const fruits = [🍑, 🍏, 🥑, 🍊, 🍉];
I'm looking for the avocado🥑 Help me find it.
Sure, you could just look at the array and say, just access it at index 2 like this fruits[2]
and you'd be right. Some salt and course-ground pepper and I'd be off enjoying my avocado.
But what if you couldn't see where the avodaco is within the array?
const fruits = [🙈, 🙈, 🙈, 🙈, 🙈]
The same fruits are still in the array, but now you're blindfolded and don't get to play peek-a-boo.
I'm still hungry😋 go find my avocado🥑!
const avocado = fruits.find((fruit) => fruit === 🥑);
All right, that was fast. Let's talk about it.
Finding the avocado🥑 is the core problem in all of computer science
If you had to think of a really difficult problem for a computer to solve, you'd come up with things far more interesting than what I'm about to tell you.
When I was a student myself, the professor told us that the single most important problem in computer science - was search.
And I was in disbelief.
He used to joke a lot, so I figured he had to be kidding. Not for a second did I believe that search could possibly be a problem let alone the most important one. Just, no way.
Oh, well.
What you need to know about arrays, and what my younger self didn't yet know, is that the computer has no clue where it stores anything😶.
You: Hey Array, take my avocado🥑 Thanks.
Instant amnesia. The array doesn't even remember what it just stored.
You: Erhm, Array, where's my 🥑?
Array: Don't know. Go search for it.
That's how arrays work.
Retrieving elements from a list requires you to loop through the list, one element at a time, and inspect each element to see🐵 which one you're currently iterating over.
The array is forgetful in the sense that it doesn't make note of where it stores any given item. You may be aware of where you stored an item, for example by pushing a pineapple🍍onto the list. Now it's the last item at the very end. You know that. But your knowledge is external to the array; it's not part of the array.
All the array knows is how many entries it has in total. And if you want to find a specific item among all entries you need to search for that item.
Every time you want to do anything with an individual item you'll have to loop through the list, look at the item you're currently sitting at and decide if that's the item you're looking for.
So, did you find the 🥑?
Keep it. It's yours.